What is service discovery in microservices?
Lets assume we have created and deployed a microservice. In order to reach microservice we need to connect with its IP address and port number. As long as the microservice is alive we can communicate with the same IP address and port numbers. This seems to have no issues until we start deploying this microservice in a virtual environment (docker containers or any cloud servers). Now if you start creating instance of the microservice, you will get a new IP adderss and port number. Everytime you redeploy you will get a different IP address. So now the location of microservice is dynamic and we still need to build a system that can interact with these microservices.
One way to achieve this is to implement a DNS server where we can maintain a hostnames and its IP addresses. But in DNS we have to manually log these new IPs in HOSTNAME.TXT file.
This is the reason we have service discovery mechanism where we ask microservices to register their location in a central server, each time they are instatiated. In addition to this we also ask microservices to ping to these central registry about its health in certain interval of time.
Example
Below is an example which explains how service registry and discovery works.
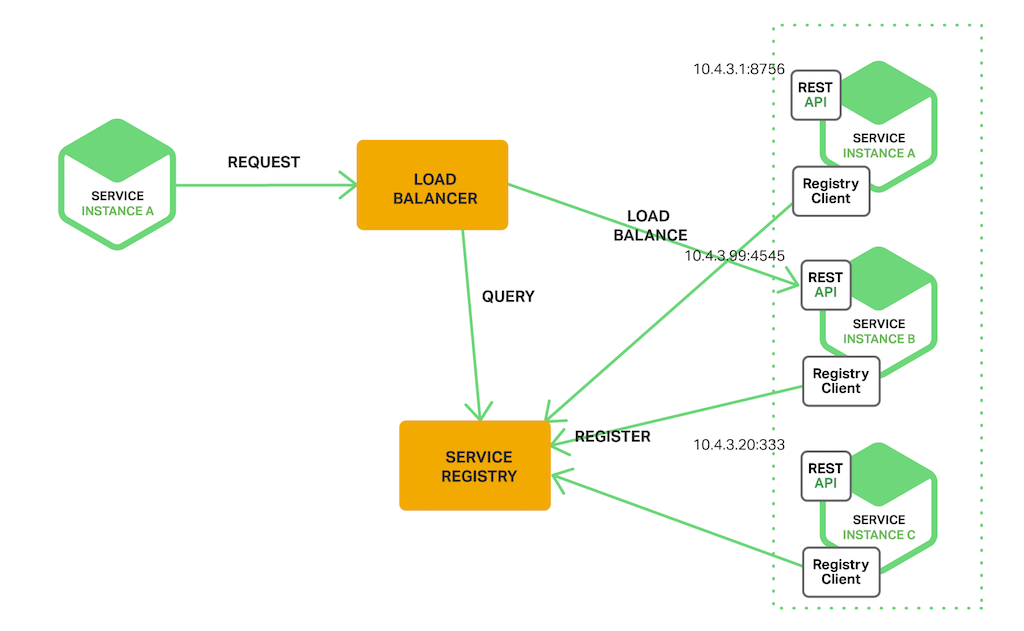
Service Registry & Discovery
In the image we have rest microservice in the right side which has its IP addresses. Each microservice has a Registry Client (e.g. Eureka Client which is provided by Netflix) which REGISTERS its IP address in SERVICE REGISTRY (e.g. Eureka Server which is provided by Netflix).
Now the SERVICE REGISTRY has all the IP addresses of microservices.
Whenever a SERVICE tries to communicate with microservice it will go through the LOAD BALANCER which makes a QUERY to SERVICE REGISTRY to get actual IP address of the microservice and then the LOAD BALANCER connects to particular microservice.
Code
Below is end to end example of achieving service-registration and service-discovery using Eureka library in Spring Boot
Run Eureka Server
We will use Eureka library to act as a service registry for our microservices. Follow below steps to setup and run Eureka server.
- Go to url: https://start.spring.io/
-
Choose dependencies as Spring Web and Eureka Server.
- Click on 'Generate button' to download a zip file. Unzip the file.
-
Import this folder into IntelliJ or Eclipse as a maven project.
-
Annotate your main class with
@SpringBootApplication
and@EnableEurekaServer
-
Change your
application.properties
file as shown below.
-
Perform maven clean install with command :
mvn clean install
-
Run the project with IDE or with command :
java -jar 'JAR_FILE_NAME'
-
Now your Eureka server will be up and running and you can view the dashboard with URL as : http://localhost:8761/
Notice that in 'Instances currently registered with Eureka' section you will find no registered microservices till now.
Run Eureka Client / Register your Microservices
We will use Eureka library to act as a service registry for our microservices. Follow below steps to setup and run Eureka server.
- Go to url: https://start.spring.io/
-
Choose dependencies as Spring Web and Eureka Client.
- Click on 'Generate button' to download a zip file. Unzip the file.
- Import this folder into IntelliJ or Eclipse as a maven project.
-
Annotate your main class with
@SpringBootApplication
and@EnableEurekaClient
-
Perform maven clean install with command :
mvn clean install
-
Run the project with IDE or with command :
java -jar 'JAR_FILE_NAME'
-
Now your microservice will be up and running and would have registered with Eureka Client.
You can go to http://localhost:8761/ and see that your microservice is now apearing in dashboard with the generated IP address.
With this IP address LOAD BALANCERS can communicate with your microservice.
Summary
Congratulations you have successfully created a Eureka Registry Server and also created a Microservice that registered itself with the server.
If you want to download the project please follow below git repositories: